Project in which this solution is applied: The Senzagro mobile app
Problem
When the user opens the mobile app, the app should fetch the latest version in the play store and compare the current app version and alert the user to update the app if the current version is behind the newest version.
The initial approach was to use the react-native-version-check library. But after a few weeks of implementing it, the feature did not work. There was a problem with the react-native-version-check library since the library fetches the SenzAgro app static HTML page in the google play store and extracts the version. However, recently google play store updated its web page. Hence to view the version the user should click a button as shown below,.
In the following static page, the version of the app is not visible
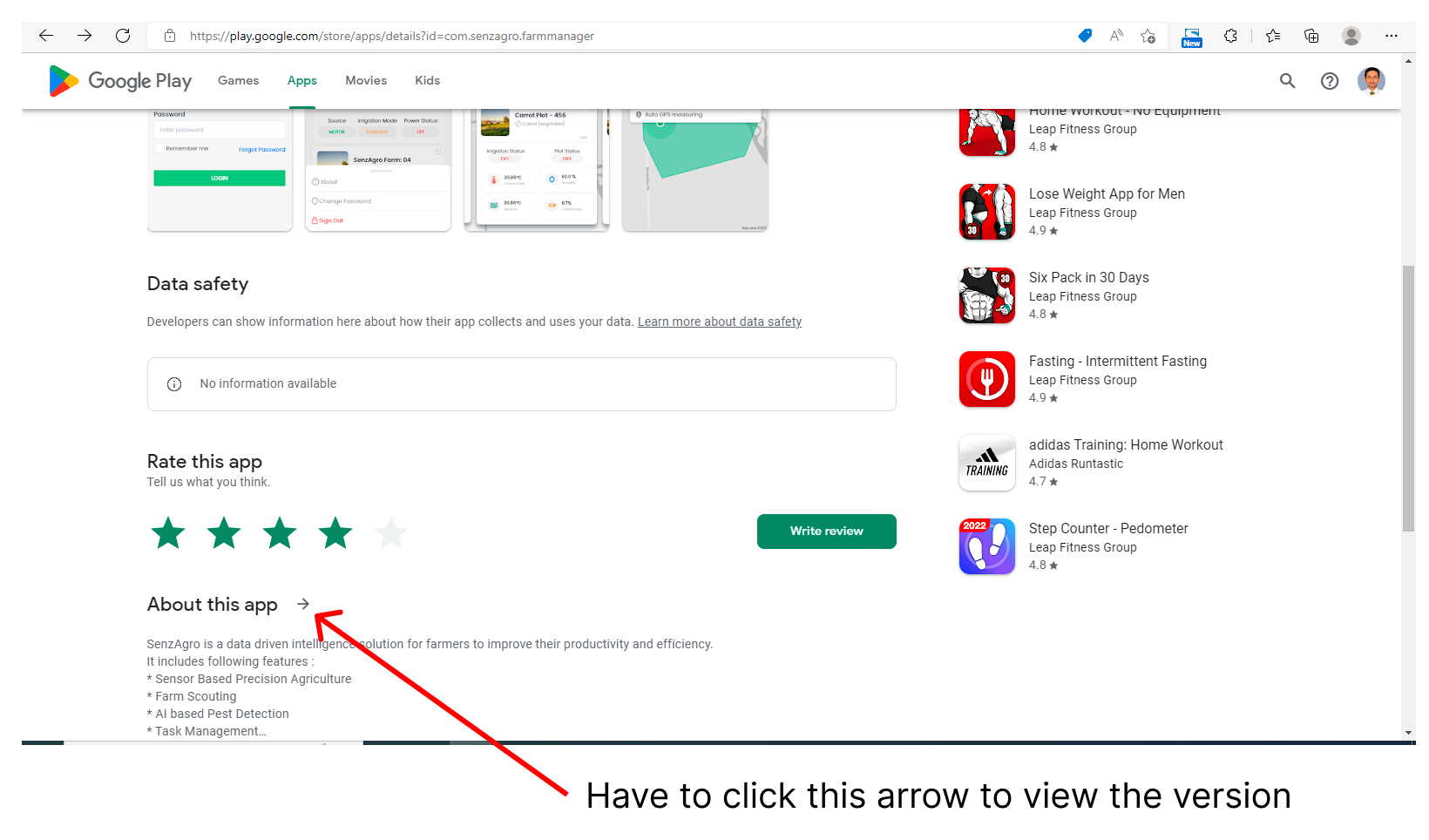
When we click the arrow the following component loads under the same URL. Thus it is not possible to fetch the version by fetching the static page from the given URL.
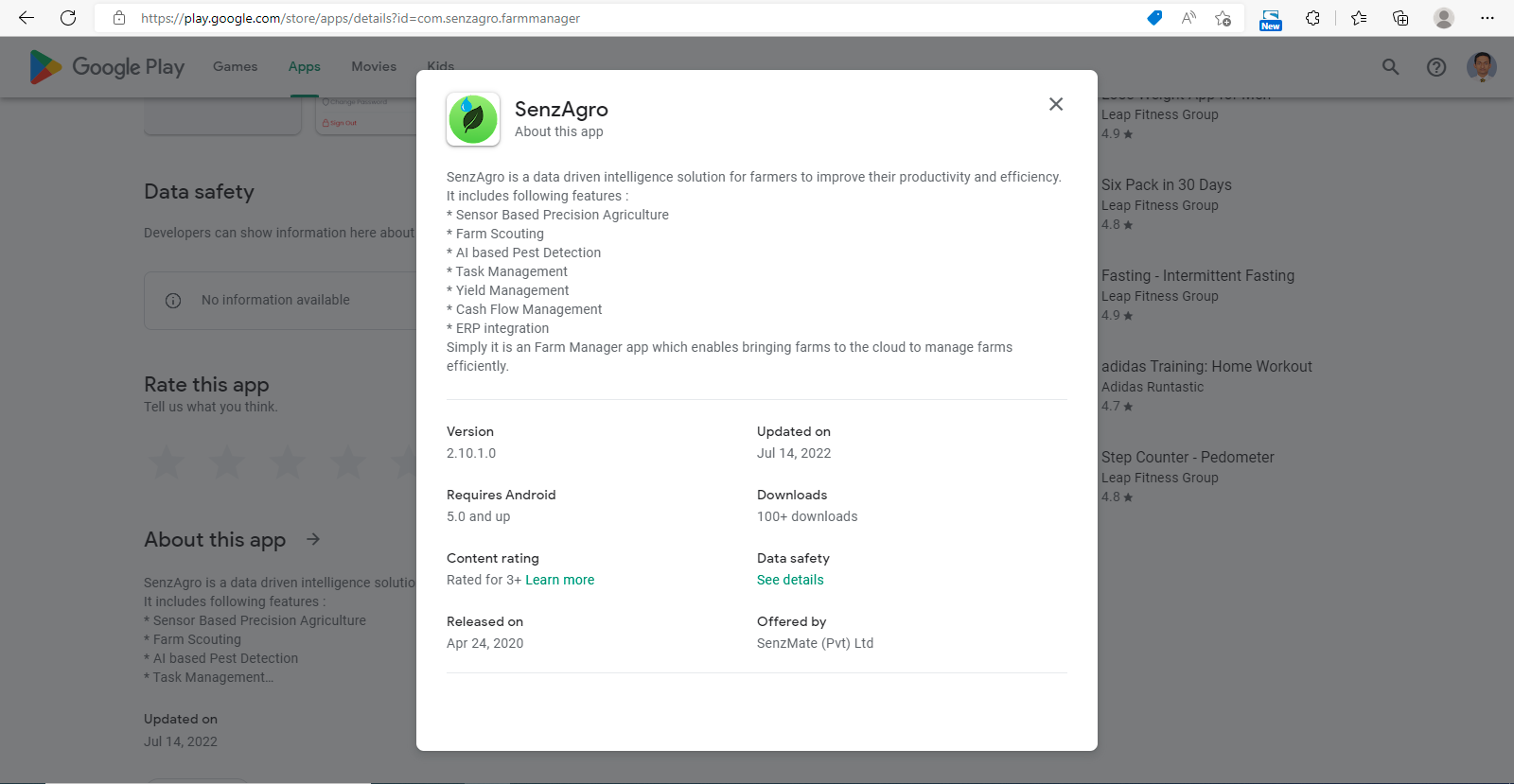
Solution
Since we wanted to find a solution for the above, we found an alternative approach to use a web driver to load the above URL in a headless-google chrome browser and click the arrow using the CSS selector of the arrow. This approach was implemented in our SenzAgro-backend by using an API.
Technical Implementation
- Programming language: Java
- The framework used: Selenium(A open-source automated testing framework)
- Backend technology: Spring-boot
STEPS
The following are the main steps that need to be followed to achieve the goal of getting the updated appVesion tag
1. Add the following Maven dependency
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>3.141.59</version>
</dependancy>
2. Download the Google chrome driver from the following website, which matches your current browser version
3. Specify the location of the web driver which you downloaded
4. The initialisation of the Chrome Driver
options.addArguments("--headless", "--disable-gpu", "--window-size=1920,1200", " --ignore-certificate-errors");
driver = new ChromeDriver(options);
driver.manage().window().maximize();
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
5. Open the headless browser and navigate to URL
6. Find the arrow button
"#yDmH0d > c-wiz.SSPGKf.Czez9d > div > div >
div.tU8Y5c > div.wkMJlb.YWi3ub > div > div.qZmL0 >
c-wiz:nth-child(2) > div > section > header > div >
div:nth-child(2) > button"));
7. Click the button
8. Wait until the dynamic component loads
wait.until(ExpectedConditions.visibilityOfElementLocated(By.cssSelector("#yDmH0d >
div.VfPpkd-Sx9Kwc.cC1eCc.UDxLd.PzCPDd.HQdjr.VfPpkd-S x9Kwc-OWXEXe-FNFY6c > div.VfPpkd-wzTsW > div > div >
div > div > div.fysCi > div.G1zzid > div:nth-child(1) >
div.reAt0")));
9. Find the div tag containing the version
div.VfPpkd-Sx9Kwc.cC1eCc.UDxLd.PzCPDd.HQdjr.VfPpkd-Sx9Kwc-OWXEXe-FNF
Y6c > div.VfPpkd-wzTsW > div > div > div > div > div.fysCi > div.G1zzid >
div:nth-child(1) > div.reAt0"));
String appVersion= version.getText();
Now you can compare appVersion with the existing version to identify whether the current app is the latest one and if not you can trigger a pop-up for the user to update the app.